Imager X Named Transforms Cache Warmer
Always keep a fresh cache of your Imager X Pro image transforms.
Dec 28, 2022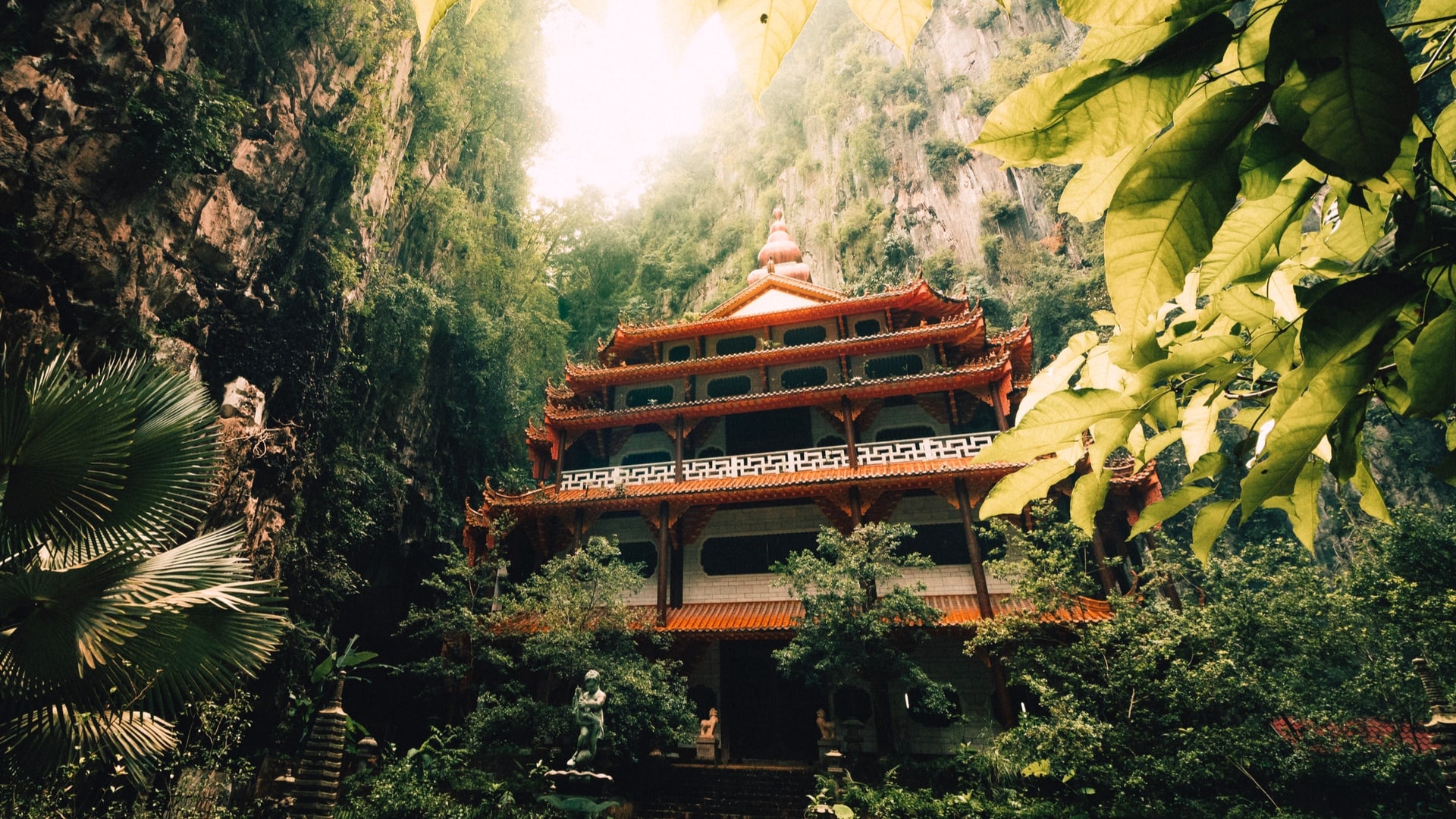
Getting Started
In this article, you will learn how to periodically generate Imager X named transform caches using a cron job.
Requirements:
Imager X Pro
Imager X Pro is great when using CraftCMS.
It allows the generation of image transformations on asset upload or element save and provides utilities in the control panel to generate named transforms on a volume level.
Configure a Named transform
# config/imager-x-transforms.php
<?php
return [
'defaultImageTransform' => [
'displayName' => 'Default Image Transform',
'transforms' => [
['width' => 400],
['width' => 850],
['width' => 1200],
],
];
];
Configure Automatic generation of transforms
# config/imager-x-generate.php
<?php
return [
'volumes' => [
'images' => ['defaultImageTransform'],
]
];
Image transformations will now be generated for the images volume on asset upload.
But what if the cache is cleared or expired?
Well, that would be an issue...
The last thing we want is for our users to experience a high TTFB. It negatively affects the UX and can increase bounce rates.
How can we prevent a missed cache and a high TTFB?
Custom CraftCMS Controller and cron job to the rescue!
Event::on(
UrlManager::class,
UrlManager::EVENT_REGISTER_SITE_URL_RULES,
function (RegisterUrlRulesEvent $event) {
$event->rules['/api/generate-named-volume-transforms'] = 'site-module/default/generate-named-volume-transforms';
}
);
<?php
namespace modules\sitemodule\controllers;
use modules\sitemodule\SiteModule;
use Craft;
use craft\helpers\App;
use craft\web\Controller;
use yii\web\Response;
use spacecatninja\imagerx\ImagerX;
class DefaultController extends Controller
{
protected array|int|bool $allowAnonymous = ['generate-named-volume-transforms'];
public function actionGenerateNamedVolumeTransforms(): Response
{
$key = Craft::$app->request->getQueryParam('key', '');
if ($key !== App::env('MODULE_API_KEY')) {
return $this->asJson([
'success' => false,
'message' => 'Invalid API Key',
]);
}
$volumeIds = Craft::$app->getVolumes()->getAllVolumeIds();
try {
ImagerX::$plugin->generate->generateByUtility($volumeIds);
} catch (\Throwable $throwable) {
$errorMessage = 'An error occured when trying to generate transform jobs from /api/generate-named-volume-transforms';
Craft::error($errorMessage . ': ' . $throwable->getMessage(), __METHOD__);
return $this->asJson([
'success' => false,
'message' => $errorMessage,
'errors' => [
$throwable->getMessage(),
],
]);
}
return $this->asJson(['message' => 'Generating ImagerX Named Volume Transforms']);
}
}
Imager will only generate the transforms that are missing or which cache has expired, and these are all done via queue jobs.
Creating the cron job
It's time to put our new controller action to use! Let's create a cron job that runs once a day at 1 a.m.
Depending on your server environment, the cron job set up may vary.
crontab.guru is a site to help schedule your cron jobs!
0 1 * * * root curl 127.0.0.1/api/generate-named-volume-transforms?key=MODULE_API_KEY
Wrapping up
Congratulations! You've made it to the end. You can sleep safely knowing your image transforms will stay up-to-date.